All game objects have a lot of data that needs to be processed by different systems(rendering, physics, animation, etc.). In order to do this quickly its better to segregate data relevant to each system and have each system deal with a specific component/structure holding that data.
For example, the physics system only needs to deal with a physics component that will hold data for mass, velocity, acceleration, force, collider, etc.
With that in mind, we created a simple entity-component structure that contains different components. The code snippet below shows the entity structure.
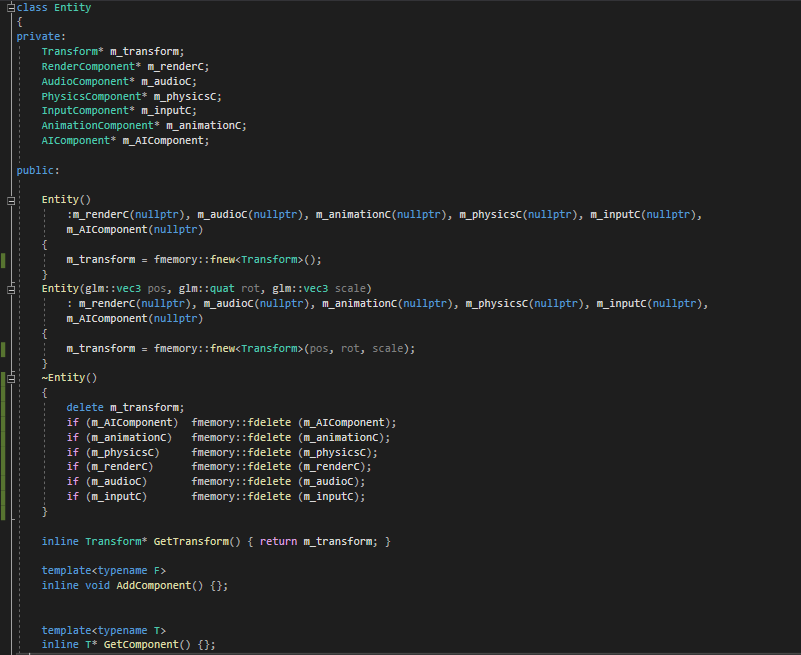
Each Entity is expected to have a transform component by default. All other components are optional. The template specializations are declared outside for compatibility with the build on Linux.
Possible Future Improvements:
Having a single component array which contains only the necessary components, which helps save on memory taken by each entity instance. This approach requires a bucket system where each component places itself in a bucket or container which is picked up by a relevant system.
Potential recreation to data-oriented design for cache-friendly access to data in a Structure-Of-Arrays format.
Interface:
A new Entity is typically created using one of our custom allocators. And a new component can be created simply by using the AddComponent Function().
For example, if a physics component needs to be created then one simply needs to use the command entity.AddComponent<PhysicsComponent>() to create a new physics component for the entity.
Similarly one may get any pre-existing component with the exception of transform component using the GetComponent() function like so:
entity->GetComponent<PhysicsComponent>();
This will return a pointer to the physics component.
The transform component needs to however be referenced using the GetTransform() function which returns to the transform component which is created by default at Entity Initialization.
Comments